치춘짱베리굿나이스
201125 따봉박는 치춘 (Discord bot) 본문
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import discord | |
client = discord.Client() | |
import asyncio | |
from discord.utils import get | |
import os | |
import random | |
token_path = os.path.dirname(os.path.abspath(__file__)) + "/token.txt" | |
t = open(token_path, "r", encoding = "utf-8") | |
token = t.read().split('-')[1] | |
client = discord.Client() | |
@client.event | |
async def on_ready(): | |
print('Logged in as') | |
print(client.user.name) | |
print('------') | |
await client.change_presence(status=discord.Status.idle, activity=discord.Game(name='👍 따봉')) | |
@client.event | |
async def on_message(message): | |
if message.author.bot: | |
return None | |
id = message.author.mention | |
channel = message.channel | |
if message.content.startswith('!따봉'): | |
if "https://discord" in message.content: | |
link = message.content.split(' ')[1].split('/') | |
if len(link) != 7: | |
await message.channel.send(id + "님! 링크가 잘못된 것 같아요") | |
else: | |
msg_id = int(link[6]) | |
msg = await channel.fetch_message(msg_id) | |
reactions = ['👍🏻', '👍🏼', '👍🏽', '👍🏾', '👍🏿', '👍'] | |
for emoji in reactions: | |
await msg.add_reaction(emoji) | |
elif "@" in message.content: | |
id1 = message.mentions[0].id | |
await message.channel.send("<@" + str(id1) + "> 님 최고! 따봉!! 👍🏻👍🏼👍🏽👍🏾👍🏿👍") | |
reactions = ['👍🏻', '👍🏼', '👍🏽', '👍🏾', '👍🏿', '👍'] | |
for emoji in reactions: | |
await message.add_reaction(emoji) | |
else: | |
t = random.randrange(7) | |
if t == 0: | |
await message.channel.send(id + "님 최고에요! 멋진 사람! 👍🏻👍🏼👍🏽👍🏾👍🏿👍") | |
elif t == 1: | |
await message.channel.send(id + "님 항상 응원하고 있죠~ 😺") | |
elif t == 2: | |
await message.channel.send(id + "님 오늘도 화이팅! 😸") | |
elif t == 3: | |
await message.channel.send(id + "님! 웃으면 복이 온대요 😄") | |
elif t == 4: | |
await message.channel.send(id + "님이 항상 행복하셨으면 좋겠어요 😉😉") | |
elif t == 5: | |
await message.channel.send(id + "님도 멋진 사람이에요 😊") | |
else: | |
await message.channel.send(id + "님! 좋은 하루 되세요!! 🥰🥰") | |
elif message.content.startswith('!나도'): | |
reactions = ['👍🏻', '👍🏼', '👍🏽', '👍🏾', '👍🏿', '👍'] | |
for emoji in reactions: | |
await message.add_reaction(emoji) | |
elif message.content.startswith('!반대'): | |
if "https://discord" in message.content: | |
link = message.content.split(' ')[1].split('/') | |
if len(link) != 7: | |
await message.channel.send(id + "님! 링크가 잘못된 것 같아요") | |
else: | |
msg_id = int(link[6]) | |
msg = await channel.fetch_message(msg_id) | |
reactions = ['👎🏻', '👎🏼', '👎🏽', '👎🏾', '👎🏿', '👎'] | |
for emoji in reactions: | |
await msg.add_reaction(emoji) | |
else: | |
await message.channel.send(id + "님은 이 의견에 반대하시나 봐요") | |
elif message.content.startswith('!하위'): | |
if "https://discord" in message.content: | |
link = message.content.split(' ')[1].split('/') | |
if len(link) != 7: | |
await message.channel.send(id + "님 하위하위~ 👋🏻👋🏼👋🏽👋🏾👋🏿👋") | |
else: | |
msg_id = int(link[6]) | |
msg = await channel.fetch_message(msg_id) | |
reactions = ['👋🏻', '👋🏼', '👋🏽', '👋🏾', '👋🏿', '👋'] | |
for emoji in reactions: | |
await msg.add_reaction(emoji) | |
await message.channel.send("하위하위~ 👋🏻👋🏼👋🏽👋🏾👋🏿👋") | |
elif "@" in message.content: | |
id1 = message.mentions[0].id | |
await message.channel.send("<@" + str(id1) + "> 님 하위하위~ 👋🏻👋🏼👋🏽👋🏾👋🏿👋") | |
else: | |
await message.channel.send(id + "님 하위하위~ 👋") | |
elif message.content.startswith('!오키') | message.content.startswith('!동의'): | |
if "https://discord" in message.content: | |
link = message.content.split(' ')[1].split('/') | |
if len(link) != 7: | |
await message.channel.send(id + "님! 링크가 잘못된 것 같아요") | |
else: | |
msg_id = int(link[6]) | |
msg = await channel.fetch_message(msg_id) | |
reactions = ['👌', '👌🏻', '👌🏼', '👌🏽', '👌🏾', '👌🏿'] | |
for emoji in reactions: | |
await msg.add_reaction(emoji) | |
else: | |
await message.channel.send("👌👌 저도 동의해요") | |
elif message.content.startswith('!펀치') | message.content.startswith('!주먹'): | |
if "https://discord" in message.content: | |
link = message.content.split(' ')[1].split('/') | |
if len(link) != 7: | |
await message.channel.send(id + "님! 링크가 잘못된 것 같아요") | |
else: | |
msg_id = int(link[6]) | |
msg = await channel.fetch_message(msg_id) | |
reactions = ['👊', '👊🏻', '👊🏼', '👊🏽', '👊🏾', '👊🏿'] | |
for emoji in reactions: | |
await msg.add_reaction(emoji) | |
else: | |
await message.channel.send(id + "님! 말보다 주먹이 빠르지만 폭력은 나빠요 💀") | |
elif message.content.startswith('!뽀큐'): | |
if "https://discord" in message.content: | |
link = message.content.split(' ')[1].split('/') | |
if len(link) != 7: | |
await message.channel.send(id + "님! 링크가 잘못된 것 같아요") | |
else: | |
msg_id = int(link[6]) | |
msg = await channel.fetch_message(msg_id) | |
reactions = ['🖕🏻', '🖕🏼', '🖕🏽', '🖕🏾', '🖕🏿', '🖕'] | |
for emoji in reactions: | |
await msg.add_reaction(emoji) | |
await message.channel.send(id + "님! 나쁜말은 안돼요! 😥😥") | |
else: | |
await message.channel.send(id + "님! 나쁜말은 안돼요! 😥😥") | |
elif message.content.startswith('!박수'): | |
if "https://discord" in message.content: | |
link = message.content.split(' ')[1].split('/') | |
if len(link) != 7: | |
await message.channel.send(id + "님에게 모두 박수 !!!! 👏👏🏻👏🏼👏🏽👏🏾👏🏿") | |
else: | |
msg_id = int(link[6]) | |
msg = await channel.fetch_message(msg_id) | |
reactions = ['👏🏻', '👏🏼', '👏🏽', '👏🏾', '👏🏿', '👏'] | |
for emoji in reactions: | |
await msg.add_reaction(emoji) | |
elif "@" in message.content: | |
id1 = message.mentions[0].id | |
await message.channel.send("<@" + str(id1) + "> 님에게 모두 박수 !!!! 👏👏🏻👏🏼👏🏽👏🏾👏🏿") | |
else: | |
await message.channel.send(id + "님에게 모두 박수 !!!! 👏👏🏻👏🏼👏🏽👏🏾👏🏿") | |
elif message.content.startswith('!슬픔'): | |
if "https://discord" in message.content: | |
link = message.content.split(' ')[1].split('/') | |
if len(link) != 7: | |
await message.channel.send(id + "님! 링크가 잘못된 것 같아요") | |
else: | |
msg_id = int(link[6]) | |
msg = await channel.fetch_message(msg_id) | |
await msg.add_reaction('😭') | |
else: | |
await message.channel.send(id + "님 울지마세요 😢") | |
elif message.content.startswith('!깔깔') | message.content.startswith('!웃음'): | |
if "https://discord" in message.content: | |
link = message.content.split(' ')[1].split('/') | |
if len(link) != 7: | |
await message.channel.send(id + "님! 링크가 잘못된 것 같아요") | |
else: | |
msg_id = int(link[6]) | |
msg = await channel.fetch_message(msg_id) | |
await msg.add_reaction('😂') | |
else: | |
await message.channel.send("웃으면 복이 와요! 그쵸 " + id + "님? 😂" ) | |
elif message.content.startswith('!흠'): | |
if "https://discord" in message.content: | |
link = message.content.split(' ')[1].split('/') | |
if len(link) != 7: | |
await message.channel.send(id + "님! 링크가 잘못된 것 같아요") | |
else: | |
msg_id = int(link[6]) | |
msg = await channel.fetch_message(msg_id) | |
await msg.add_reaction('🤔') | |
else: | |
await message.channel.send("흠.. 그러게요 " + id + "님.. 🤔") | |
elif message.content.startswith('!졸작'): | |
reactions = ['👎🏻', '👎🏼', '👎🏽', '👎🏾', '👎🏿', '👎'] | |
for emoji in reactions: | |
await message.add_reaction(emoji) | |
await message.channel.send("졸작은 나쁜 문명 😡") | |
elif message.content.startswith('!부트'): | |
await message.channel.send("사차원 ~ 😄") | |
elif message.content.startswith('!시공'): | |
await message.add_reaction('🌀') | |
t = random.randint(0, 2) | |
if t == 0: | |
await message.channel.send("🌀시공의 폭풍으로 어서오세요🌀") | |
elif t == 1: | |
await message.channel.send("🌀시. 공. 좋. 아.🌀") | |
else: | |
await message.channel.send("🌀https://heroesofthestorm.com/ko-kr/🌀") | |
elif message.content.startswith('!귀여워'): | |
await message.add_reaction('😄') | |
await message.channel.send("감사합니다 😄😄") | |
elif message.content.startswith('!사랑해'): | |
reactions = ['❤️','🧡', '💛', '💚', '💙', '💜', '🖤', '🤍', '🤎', '💗'] | |
for emoji in reactions: | |
await message.add_reaction(emoji) | |
await message.channel.send("많은 사랑 주셔서 정말 감사합니다 " + id + "님! 😄") | |
client.run(token) |
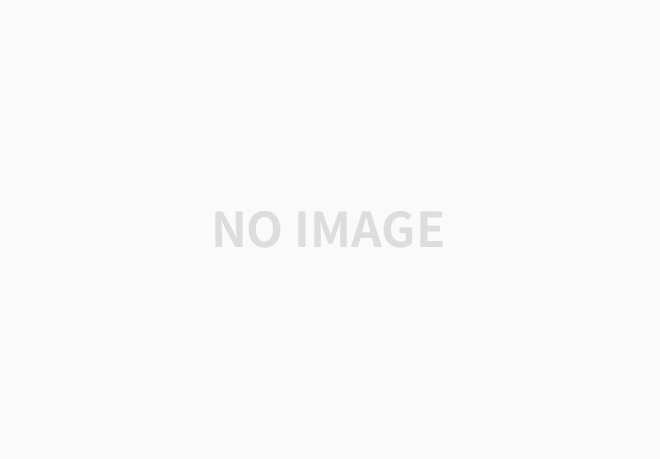
디스코드 할때 상대방한테 이모티콘 달아주는게 번거로와서 봇을 하나 만들었다
이름하야 따박치
블로그에 올리는이유는 : 공부했던것들 깃헙에 백업하면서 깃 만지다가 실수로 디코봇 토큰까지 올려버렸는데
히스토리까지 없앤다고 git reset --hard (커밋명) 한다음에 git push -f origin master (강제 푸시) 했더니 파일이 완전히 날아가서
git reflog으로 커밋 되살려서 어찌어찌 부활시킴... 깃을 공부합시다
'Python > 기타' 카테고리의 다른 글
200720 음성 파일 Text 변환 (feat Google STT) (0) | 2021.02.08 |
---|---|
200720 bin -> RGB json / csv 변환기 (0) | 2021.02.08 |
191123 OpenCV 갖고놀기 (0) | 2021.02.07 |